Cloud Task Parallelization in .NET
Research Project: 2012 - 2016.
Cloud Task Parallelization enables a seamless distribution of tasks in .NET on parallel computing resources in the cloud, such as on high-performance computing clusters. By way of a new .NET runtime extension, programmers can directly invoke a set of tasks for automatic distribution and scheduling on remote processors. The distributed tasks are programmed equivalently to conventional .NET tasks, each expressed as an ordinary .NET delegate (or lambda). The runtime system automatically collects the reachable task code and data and ships this to computing resources into the cloud. On the cloud side, the tasks are consequently recompiled and executed on a potentially large amount of cores or nodes. The results of the remote task computation are eventually sent back to the client and also its side-effect changes made effective in memory. In the current stage, the system is realized for .NET as client-side runtime and MS HPC as a server-side platform.
Programming Model
Programming with distributed tasks is mostly analogous to using conventional .NET TPL tasks. As only prerequisite, the .NET library HSR.CloudTaskParallelism.Client.Runtime
needs to be referenced - no extra compile step is necessary.
Distributed tasks are described as ordinary .NET delegates (or lambdas). They must be isolated against each other and also against other threads in the program, i.e. they access only disjoint fields or array elements, except for read-only accesses.
A set of distributed tasks can be started on remote processor resources in one bunch to reduce network roundtrip costs. The termination of a task can be awaited by the Wait()
method or by reading the Result
property of a task.
For running distributed task, an instance of the class Distribution
has to be created by specifying the service URI and authorization token (password/secret) for the task parallelization web service.
public void FactorizeNumbers(long[] inputs) {
var distribution = new Distribution("http://tasks.concurrency.ch", "authorizationtoken");
var taskList = new List<DistributedTask<long>>();
foreach (var number in inputs) {
var task = DistributedTask.New(
() => Factorize(number)
);
taskList.Add(task);
}
distribution.Start(taskList);
foreach (var task in taskList) {
Console.WriteLine(task.Result);
}
}
private long Factorize(long number) {
for (long k = 2; k * k <= number; k++) {
if (number % k == 0) { return k; }
}
return number;
}
Distributed parallel loops allow an even simpler description of the same scenario (similar to the TPL Parallel.For
):
distribution.ParallelFor(0, inputs.Length, (i) => {
outputs[i] = Factorize(inputs[i]);
});
Runtime system
The client runtime library is in charge of automatically extracting all reachable code and data (objects and static fields) of the distributed tasks and shipping this information to the task parallelization web service. Consequently, the web service dispatches the task invocations to remote processing resources, e.g. a MS HPC cluster. On execution nodes, the task code is recompiled and its data deserialized before the tasks are instantiated and started. When terminated, the results and all side-effect changes of the tasks are collected, serialized and transported back to the client, where the changes are finally made effective in local memory. The runtime system even detects illegal write-write conflicts among distributed tasks in the case that programmers violate the task isolation.
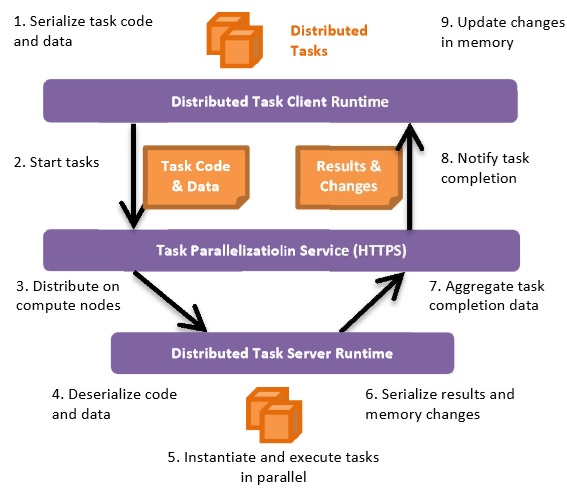
Download (Client Components)
Download (Server Components)
Related Publication
- L. Bläser. Task Parallelization as a Service: A Runtime System for Automatic Shared Task Distribution. Eight Workshop on Programmability Issues for Heterogeneous Multicores (MULTIPROG-2015) at HiPEAC 2015, Amsterdam, The Netherlands, Jan. 2015.
Presentation.
- L. Bläser. .NET Task Parallelization in the Cloud: .NET Task Parallelization in the Cloud: Runtime Support for Seamless Distribution of Shared Memory Parallel Tasks. In 4th Workshop on Systems for Future Multicore Architectures (SMFA'14) at Eurosys 2014, Amsterdam, Netherland, Apr. 2014.
Paper.
License and Legal Disclaimer
Please pay attention to the license and legal disclaimer (click here) for the documents and software of the programming language and system.